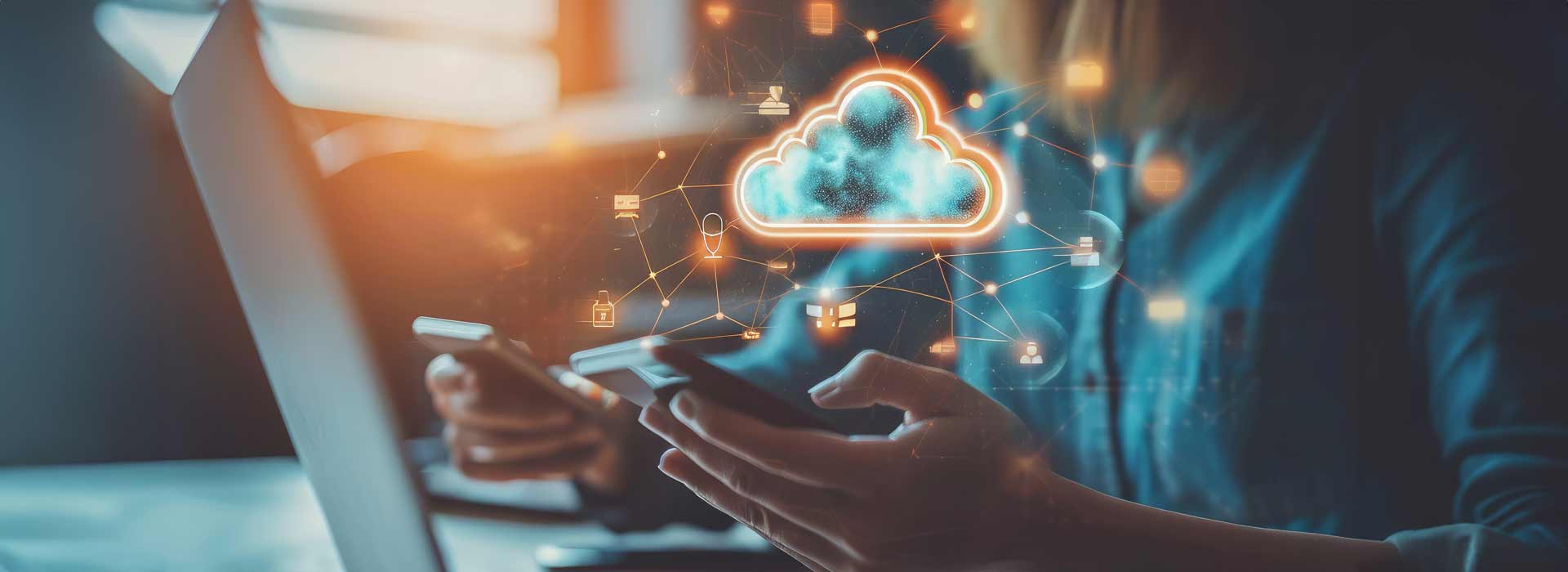
- Akira Haruka
Redux State Management for React: A Comprehensive Guide
Microservice architecture is the new trend in the modern age of front-end development. The approach involves splitting various data flows/ separating the concerns of your application into the components. While this introduces many benefits and creates a degree of simplicity, it can also create complexity when several components depend on the same data.
React is a front-end library. Redux is managing the application’s state holds to a single global object called the store, and solves where the props need to be passed deep down into the component tree which is also called the ‘prop drilling’ problem. Redux’s fundamental principles help in maintaining consistency throughout the application, which makes debugging and testing easier.
Redux is a simple JavaScript library that helps to manage states in any JavaScript-based application such as React, Angular, Vue, etc.
Prerequisite:
- Familiarity with React core concepts
- Familiarity with async JavaScript
What is Redux?The Redux pattern has several defining characteristics:
- The state of your whole application is stored in an object tree within a single store (Single source of truth).
- The store is immutable (it cannot be directly changed – instead changes create a new copy of the store).
- Actions are dispatched on a shared message bus, handled by pure functions called reducers. Reducer is a pure function that takes state and action as arguments, returning a new state from the initial state.
REDUX data flow
The following figure shows the data flow of Redux state management:
View
Let us understand what is application state management and the different types of application states first. State management is the idea of sharing application data across multiple components within the same application where each component can read and modify the application state depending on the requirement. We have been emitting the event from here.
React component uses application state to render the portions of UI which have undergone changes without refreshing the complete page. The application state can be divided into two:
- Local state – it’s the state which is used only inside a particular component and not being shared across any other components.
- Global state – it’s the state which is being initialized in one parent component shared across multiple components which can be a direct child or part of a different component tree.
We can get the data from the store and the state gets the instantiation of the selector into the use Selector method from react-Redux library.
Import {use Selector} from ‘react-Redux’;
const username = useSelector(({auth}) => {
Return auth. Username
)
Action
Action is a simple JavaScript object that is used to send (dispatch) information from React components to other building blocks. It describes all the changes in the state of the application after the user interaction, internal events, and API calls.
Here’s the code of the Action object
{
type: “USER_LOGIN”,
Payload: {username: “John Doe”, password: “123456”}
}
Action must have ‘type’ property and optional payload data which should be communicated to other blocks. Action payloads can be read by reducers.
We can call the action object with the instantiation dispatch of the use Dispatch method from the react-Redux library
import {use Dispatch} from ‘react-Redux’Const dispatch = useDispatch()
//dispatching to the action creators functions
dispatch(authLogin(userCred))
Reducers
Reducers read the payloads from the actions and then update the store accordingly. It’s a pure function to return a new state from the initial state.
Function authReducer(state, action) {
switch (action.type) {
case ‘USER_LOGIN’:
return {…state,
auth: action. payload
}
default:
return state
}
}
Store
A store is an immutable object tree in Redux. A store is a state container that keeps the application’s state. Redux can have only one store in your application. Whenever a store is created in Redux, you need to specify the reducer. UI will be rendered automatically when the subscribe a selector call-back in a Redux store.
The store is created using the creator.
Store method from the Redux library
import {createStore } from “redux”;const store = createStore(authReducer)
You can pass it to the reducer function as a parameter. Now you are ready to dispatch an action that is managed by the reducer:
let userCred =
{ username: “johndoe”, password: ‘123456’ };
store.dispatch(authLogin(userCred));
The finalization of providing the store to be available for your application to our react components by putting a React Redux in the src/index.js file.
We can create the Provider selectors from the React-Redux library.
import { Provider } from ‘react-redux’import AppView from ‘./AppView’;
import { store } from ‘./store’
const App = () => {
return (
)
}
export default AppThis is how we start state management with React applications using React and React-Redux.
Conclusion
Redux is one of the most used libraries in state management for React applications. Redux has a lot of middleware libraries available in React such as Redux-Saga and Redux thunk, it’s especially used in handling API calls and side effects. We can write a standard way to Redux logic using ReactJS/toolkit. I hope this article will be a good first step in creating your own React application using Redux, React-Redux.